How to build a Residential Proxy Pool
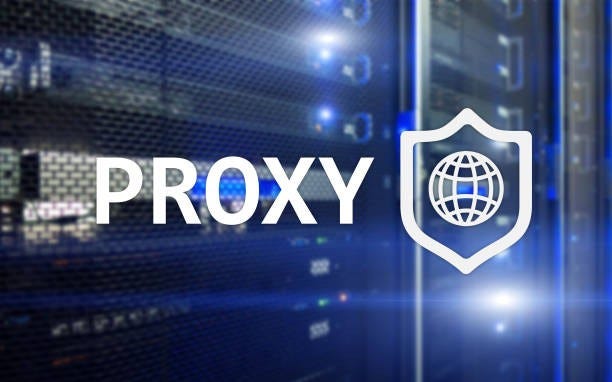
Here’s a quick confession: when I first stumbled upon the phrase “residential proxy pool,” I assumed it was some hush-hush corner of the internet that only secret agents or superhackers knew about. Turns out, it’s not quite CIA-level intel, but it’s definitely a power move for anyone who wants to manage traffic, scrape websites, or run any number of data-driven tasks with a bit of anonymity. Think of it as the big kid’s version of a typical proxy solution — one that taps into real, everyday home connections across the globe instead of the all-too-familiar (and sometimes suspicious) data-center IPs.
So, if you’ve ever tried to do large-scale web scraping or bypass region locks only to be blocked by your target site, a residential proxy pool might be your new best friend. Let’s dive deep into how to build one, mixing in a little anecdotal flair, a dash of technical steps, and a friendly warning here or there. Because if there’s anything I’ve learned from investigating questionable browser extensions or random “free” deals, it’s that you always want the real story — and a good plan.
Why Even Bother with a Residential Proxy Pool?
Because standard proxies can be a big old bull’s-eye on your back. Many websites instantly recognize data-center IPs and do their best to block them. Residential proxies (i.e., IP addresses tied to actual homes or devices) are trickier to detect. You look more like a normal user in your pajamas watching cat videos than a sophisticated scraping script or a suspicious spammer.
In essence, you get:
• Better success rates.
• Fewer blocks or captchas.
• More trust signals to target sites.
That said, you can’t just snap your fingers and conjure up a massive pool of real IPs overnight. It takes planning, tech know-how, and a healthy respect for the folks whose connections you’re about to route through.
Residential Proxy Pool 101: The Core Ingredients
1. A Source of Residential IPs
The heart and soul of a residential proxy pool is real user IP addresses. You can’t just spin them up on demand like a cloud instance; they have to come from ISPs that serve real houses. Some folks do this by partnering with third-party providers who manage large networks of willing participants — often in exchange for something (a discount, a free tool, or ironically, a “free money” promise that might taste as sweet as honey but comes with hidden strings).
2. Under-the-Hood Infrastructure
You’ll need servers to manage traffic, route requests, and keep track of uptime. Think of these servers as your personal traffic cops, directing internet flows from your pool of residential IPs to your end destination.
3. Robust Routing and Load Balancing
Once you have your pool of IPs, you have to decide how to rotate them. Randomly? Round-robin? Weighted by region? If you’re scraping, you probably want frequent rotations (so you don’t appear to be that single user requesting 10,000 pages in a minute). If you’re managing region-based ads, you might want stable sessions from a particular location.
4. A Dash of Monitoring and Logging
If you’re not logging or monitoring traffic, you might miss critical data: which IP is blacklisted, which region has the highest latency, or whether someone on your network is up to no good. Think of it like having security cameras in a parking lot — if something fishy happens, you’ll want to review the footage.
5. A Thorough Understanding of Legal/Ethical Implications
Residential IPs belong to real people. This isn’t purely about spinning up ephemeral machines in a faraway data center. You must ensure everything’s above board: consent, usage terms, local laws, all that stuff that keeps you from starring in a cautionary tale on the nightly news.
The Basic Blueprint for Building Your Own Residential Proxy Pool
Step 1: Gather IPs (Without Hijacking Anyone’s Connection)
• Option 1: Enrollment Programs
Some companies run user-consent programs. Participants opt in to share idle bandwidth in exchange for something (like gift cards, free software, or yes, sometimes a shady-sounding “browse and earn” offer). If you’re building a pool on your own, you could design a small-scale version. For instance, you build a legit app or browser extension that users willingly install, and they get perks in return.
• Option 2: Partnerships with Established Providers
The simpler path: partner with an existing residential proxy provider who already has a network of participating devices. Typically, you pay a fee, get an API or interface, and incorporate it into your system. You skip the hassle of building your own user base.
Step 2: Set Up the Server Architecture
• Reverse Proxy Server
This is the brains of the operation. It accepts your requests (maybe from a web-scraping script), then decides which residential IP to use. You might code this in Node.js, Python, or Go — pick your poison.
• Database or Cache Storage
You need some way to keep track of your IP list: which ones are online, offline, or blacklisted. A fast cache like Redis can do wonders.
Step 3: IP Rotation Logic
• Random Rotation vs. Sticky Sessions
– Random rotation means every new request uses a different IP from the pool. Great for anonymity, not so great for tasks requiring a login.
– Sticky sessions keep you on the same IP for a set duration or number of requests. This is handy if you need to stay logged in or not raise eyebrows by “moving” all over the world every time you load a new page.
• Frequency of Rotation
Decide how long an IP is assigned. Rotate too fast, and the site might smell something fishy. Rotate too slow, and you might get flagged as a suspiciously active single user.
Step 4: Logging, Monitoring, and Alerts
You can’t just “fire-and-forget” a residential proxy pool. You’ll want real-time alerts for:
• IP conflicts or duplications.
• Abnormal 404 or 403 error rates (sites are blocking you).
• Latency spikes or timeouts.
That’s where a quick logging approach comes in. For example, you might use an ELK (Elasticsearch, Logstash, Kibana) stack or a simpler solution if you’re on a budget. The moment your logs show a cluster of 403 errors from a particular region, you know it’s time to rotate or temporarily remove that batch of IPs.
Step 5: A Brief Code Sample (A Tiny Peek Under the Hood)
Below is a rudimentary Node.js snippet that demonstrates how you might route requests through different IP endpoints (assuming you already have a list of residential IPs and ports). Keep in mind, in a real production scenario, you’ll integrate more robust error handling and dynamic IP rotation:
Simple IP rotation using Node.js http-proxy
const http = require(‘http’);
const httpProxy = require(‘http-proxy’);
// Suppose we have an array of available IPs
// Each entry is an object: { host: ‘xxx.xxx.xxx.xxx’, port: 1234 }
const residentialPool = [
{ host: ‘192.168.1.10’, port: 8080 },
{ host: ‘73.22.14.55’, port: 8081 },
{ host: ‘45.67.88.90’, port: 9090 },
// …and so on
];
const proxyServer = httpProxy.createProxyServer({});
let currentIndex = 0;
const server = http.createServer((req, res) => {
// Round-robin approach
const target = residentialPool[currentIndex];
currentIndex = (currentIndex + 1) % residentialPool.length;
proxyServer.web(req, res, { target: `http://${target.host}:${target.port}` }, (error) => {
console.error(‘Proxy error:’, error);
res.writeHead(500);
res.end(‘Something went wrong.’);
});
});
server.listen(3000, () => {
console.log(‘Residential proxy pool running on port 3000’);
});
——————————————————————————–
In this example, we rotate through the residentialPool array on each request. A real-world scenario might incorporate sticky sessions or more advanced logic based on error codes and user needs.
Potential Pitfalls and How to Avoid Them
• Legal Quicksand
Always confirm you have consent or the right to use someone’s bandwidth. Even if you get that from a third-party vendor, read their terms carefully. The last thing you want is to find out it was more like a “Honey” approach: so-called free, but actually ripping someone off.
• Over-Scraping and Getting Blocked
A “residential proxy pool” doesn’t mean “unlimited scraping heaven.” Sites that get hammered with requests will clamp down. You still need to pace yourself, mimic normal user behavior, and handle captchas gracefully.
• Performance Bottlenecks
Residential IPs can be slower than data-center IPs. They’re real broadband or Wi-Fi connections. Don’t expect blazing, symmetrical speeds for every IP. For large-scale operations, you may need a bigger pool or a level of concurrency that still keeps your overall performance in check.
• Ethical Considerations
If you’re building your own pool by persuading users to install an app or extension, be crystal clear in your disclaimers about bandwidth usage. Don’t bury it behind 15 screens of legalese. A trust-based relationship is always more sustainable than a borderline scam.
Long-Term Maintenance:
Just like how your phone constantly begs for updates, your residential proxy pool isn’t a set-and-forget project. You’ll rotate IPs, remove flagged ones, add new ones, watch your logs for suspicious spikes, and possibly renegotiate terms with your IP source. It’s a living, breathing system that reacts to changes in the real world — from evolving scraping targets to user churn if you’re running your own volunteer network.
Why This Matters (A Reality Check)
We live in an era where data is like that shiny new NFT everybody’s talking about — everyone wants it, but not everyone knows how to get it. Residential proxies can be your golden ticket to legitimate data (or in some cases, questionable data) without wearing a giant “I’m a bot!” sign. But with great power comes great responsibility. When you’re co-opting real IP addresses, you also risk real consequences if something shady goes down on your watch.
Final Thoughts:
A well-built residential proxy pool is a game-changer. But do it thoughtfully. Know the tech. Understand the legalities. Respect the people behind those IP addresses. Then, once you’ve got everything humming, watch as the dreaded “Access Denied” pages fade into a memory… or at least become less frequent. Sometimes, it can be easier to use a orebuild solution for Residential Proxies to be honest, but that is up to you. Because, let’s face it, the internet is a wild place, and we can all use a bit of help navigating it.
Remember: If a solution ever seems too good to be true or “free,” scrutinize it. The real cost might be hidden behind a paywall of mistrust or potential lawsuits. Keep your eyes open, your logs tidy, and your IPs rotating — and you’ll have a robust residential proxy pool that can open digital doors without slamming them shut on your future.