HTML CSS JavaScript: A Comprehensive Guide for Beginners and Professionals
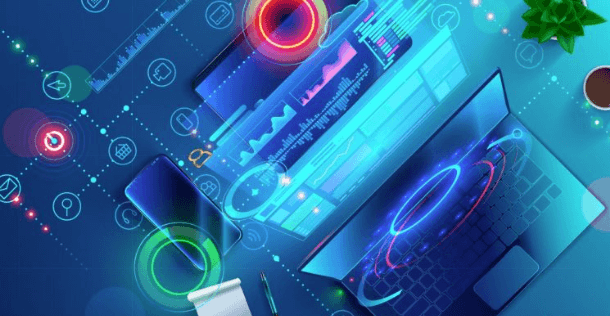
Introduction to Web Development
Web development is the process of building and maintaining websites and web applications. It involves a combination of technical skills, including programming languages, markup languages, and databases. At its core, web development is about creating functional, user-friendly web pages that provide a seamless experience across different devices and browsers.
To become a proficient web developer, you need a solid understanding of HTML, CSS, and JavaScript. These three technologies form the foundation of web development, allowing you to structure content, style it, and add interactivity. However, web development is a rapidly evolving field, and staying updated with new tools and technologies is crucial.
Web development is not just about writing code; it’s a creative field that requires problem-solving skills, attention to detail, and the ability to work well under pressure. Whether you’re building a simple blog or a complex web application, mastering HTML, CSS, and JavaScript is essential for success in this dynamic industry.
Why Learn HTML, CSS, and JavaScript?
HTML, CSS, and JavaScript form the foundation of modern web development, making them essential for anyone looking to build websites or web applications. These three technologies work together to create the structure, design, and interactivity of web pages, playing a pivotal role in user experience and functionality.
HTML (Hypertext Markup Language) is the building block of any website, defining the structure and layout of web pages. It organizes content through tags, allowing browsers to interpret and display elements such as text, images, and links.
CSS (Cascading Style Sheets) is responsible for the visual appearance of a website. By controlling fonts, colors, layouts, and spacing, CSS brings HTML to life, making websites visually appealing and responsive across different devices.
JavaScript adds interactivity to websites, enabling dynamic elements like forms, animations, and user-triggered actions. It’s what powers the rich, engaging experiences that users expect from modern websites.
Modern web browsers fully support HTML, CSS, and JavaScript, enhancing their functionality and interactivity.
Learning these three technologies opens up endless opportunities, whether you’re designing a simple blog or building complex web applications.
1. HTML: The Building Blocks of the Web
What is HTML?
HTML (HyperText Markup Language) is the foundational language used to create the structure of web pages. It defines the content of a website using a system of tags and attributes, forming the skeleton of all web pages. Unlike CSS, which is used for styling, and JavaScript, which adds interactivity, HTML establishes the basic framework that browsers interpret to display web content. Even with just HTML, you can create a functional and appealing website.
HTML consists of a series of elements represented by tags, such as < header>, < p>, and < a>, which define different types of content on a webpage. These elements often have attributes, such as href for hyperlinks, which provide additional information about the element.
Basic Structure of an HTML Document
An HTML document is structured with specific tags, including:
- <!DOCTYPE html>: Declares the document type and version of HTML.
- <html>: Encloses the entire HTML document.
- <head>: Contains metadata, links to stylesheets, and scripts.
- <body>: The main content of the webpage, such as text, images, and links.
Example:
<!DOCTYPE html>
<html lang=”en”>
<head>
<title>My Web Page</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a sample paragraph.</p>
<a href=”https://example.com”>Click here to learn more!</a>
</body>
</html>
How HTML Powers Web Development
HTML’s role in web development is crucial because it ensures accessibility and SEO friendliness. Semantic HTML, where you use meaningful tags (like < article>, < section>, and < nav>), makes the web more accessible to people using screen readers, and helps search engines understand the page content better.
HTML is a crucial part of web programming, which involves creating and maintaining websites.
Real-world application: Major websites such as Facebook and Wikipedia use well-structured semantic HTML to ensure high visibility in search engine results while maintaining accessibility.
Learning Path
- Beginner Section: Start by learning basic HTML elements such as headings (< h1> to < h6>), paragraphs (< p>), and links (< a>). Practice adding images, creating lists, and structuring content logically.
- Advanced Section: Explore HTML forms, incorporating input fields, buttons, and form validation. Dive into HTML5, which introduces multimedia elements like < audio>, < video>, and < canvas>, enabling richer web experiences.
Interactive Code Example:
< !DOCTYPE html>
< html>
< body> < h1>HTML Form Example< /h1>
< form action=”/submit-form”>
< label for=”name”>Name:< /label>
< input type=”text” id=”name” name=”name”>
< label for=”email”>Email:< /label>
< input type=”email” id=”email” name=”email”>
< button type=”submit”>Submit< /button>
< /form> < /body>
< /html>
This simple form allows users to input their name and email and submit the information.
SEO and Technical Benefits
Well-structured HTML contributes significantly to SEO, as search engines prioritize content that’s easily readable and accessible. Using tags like <h1> for headings and <p> for text helps search engines crawl and rank web pages better. Clean and semantic HTML is vital for ensuring high rankings and smooth user experiences across devices.
2. CSS: Making the Web Look Good
What is CSS?
CSS, or Cascading Style Sheets, is the language used to enhance the visual appearance of web pages. While HTML provides the structure, CSS is responsible for the design, including colors, fonts, layout, and the overall presentation of the content. By separating the content (HTML) from its presentation (CSS), web developers can control the look and feel of a website with flexibility and ease.
Cascading Style Sheets: Structure and Syntax
CSS works by applying styles to HTML elements through rules composed of selectors and declarations. For example:
p {
color: blue;
font-size: 16px;
}
In this example, the p selector targets all paragraph elements, and the declarations change the text color to blue and the font size to 16px. The cascading nature of CSS means that the styles applied to parent elements can influence child elements, offering efficiency and simplicity in design.
Applying CSS to HTML
CSS can be applied in three main ways:
- Inline CSS: Styles are applied directly within an HTML element, using the style attribute.
- Internal CSS: CSS rules are placed within a <style> tag in the <head> section of the HTML document.
- External CSS: A separate CSS file is linked to the HTML document, enabling the same styles to be applied across multiple pages.
For example, external CSS is highly recommended for better organization and maintainability.
Basic CSS Properties
CSS allows developers to control various design aspects, including:
- Colors: Define background and text colors using hex codes, RGB, or predefined color names (e.g., color: red;).
- Fonts: Specify the font family, size, and weight (e.g., font-family: Arial, sans-serif;).
- Layout: Use properties like width, margin, and padding to control the spacing and positioning of elements.
Importance of Responsive Design
In today’s mobile-first world, ensuring that websites are accessible and functional across all devices is essential. CSS enables responsive design through media queries, which allow developers to apply different styles depending on the device‘s screen size. By using flexible grid layouts, fluid images, and breakpoints, CSS ensures that websites work seamlessly on desktops, tablets, and smartphones.
Real-world application: Websites like Airbnb and Amazon leverage responsive CSS techniques to provide an optimized user experience on all devices. For instance, CSS Grid and Flexbox are essential tools for creating flexible and responsive layouts, allowing elements to resize and reposition based on screen size.
Interactive Example: Change Colors and Fonts
Try this simple interactive CSS example. You can copy and paste it into a live editor to see how CSS changes the appearance of HTML elements:
<!DOCTYPE html>
<html lang=”en”>
<head>
<style>
body {
background-color: lightgrey;
font-family: Arial, sans-serif;
color: black;
}
h1 {
color: darkblue;
}
p {
font-size: 18px;
line-height: 1.6;
}
</style>
</head>
<body>
<h1>Welcome to My Stylish Page</h1>
<p>Change the colors and fonts to see how CSS transforms the look!</p>
</body>
</html>
This example allows you to see how changing properties like color and font-family instantly affects the page’s style.
Learning Path
- Beginner Section: Learn foundational concepts like the box model, which controls how margins, borders, padding, and content are laid out. Understanding these basics is essential for controlling space and layout.
- Advanced Section: Dive into media queries, CSS Grid, and Flexbox, which are used to create responsive, flexible, and complex layouts. These advanced tools are the backbone of modern web design, helping websites adapt to different screen sizes and orientations.
3. JavaScript: Adding Interactivity
What is JavaScript?
JavaScript is a powerful programming language that adds dynamic functionality to websites. While HTML structures a webpage and CSS styles it, JavaScript enables interactivity, making websites more engaging. From form validation and animations to real-time updates and interactive maps, JavaScript powers countless features that enhance the user experience. While JavaScript is essential, understanding other programming languages can enhance your web development skills.
How JavaScript Works with HTML and CSS
JavaScript works hand-in-hand with HTML and CSS to control a website’s behavior. One of the primary ways it interacts with HTML is through DOM (Document Object Model) manipulation, allowing developers to dynamically change the content and structure of a webpage. For example, JavaScript can hide or show elements, change text, and update styles on the fly. Additionally, JavaScript is key for event handling—such as responding to user clicks or key presses—and for creating smooth animations that provide a polished, interactive feel.
Interactive Example
Let’s demonstrate the power of JavaScript with a simple example. Below is an interactive code snippet where users can click a button to change the content displayed on the page:
<!DOCTYPE html>
<html lang=”en”>
<head>
<title>JavaScript Example</title>
<style>
#content {
font-size: 20px;
color: darkgreen;
}
button {
font-size: 18px;
padding: 10px;
margin-top: 10px;
}
</style>
</head>
<body>
<div id=”content”>Click the button to change this text!</div>
<button onclick=”changeText()”>Change Text</button>
<script>
function changeText() {
document.getElementById(“content”).innerHTML = “The text has changed!”;
}
</script>
</body>
</html>
This example demonstrates basic DOM manipulation—by clicking the button, the text inside the div element changes, showcasing the simplicity and flexibility of JavaScript.
Learning Path
- Beginner Section: Start by learning basic JavaScript concepts, including variables, data types, functions, and loops. Understanding these foundations is crucial for building more complex interactions.
- Advanced Section: Once comfortable with the basics, move on to advanced topics like asynchronous programming (using async/await and promises), APIs, and modern ES6+ features like arrow functions and destructuring. These skills allow developers to build interactive web applications that fetch data and update content without reloading the page.
4. Combining HTML, CSS, and JavaScript
Why They Work Together
HTML, CSS, and JavaScript are the fundamental building blocks of web development, and their synergy is essential for creating interactive, visually appealing websites. HTML provides the basic structure of a webpage, while CSS styles the content, and JavaScript adds dynamic interactivity. This combination enables developers to build responsive, engaging websites that deliver a seamless user experience.
For example, HTML creates the structure of a form, CSS styles it, and JavaScript validates the user input in real-time. Together, these technologies allow websites like Amazon or YouTube to function smoothly, offering a blend of design and interactivity that enhances usability.
Building a Simple Web Page
Let’s go step-by-step to create a simple webpage using HTML, CSS, and JavaScript:
- HTML (Structure)
HTML sets the foundation of your webpage. For example, you can create a header, paragraph, and button with basic HTML:
<!DOCTYPE html>
<html lang=”en”>
<head>
<title>Simple Web Page</title>
</head>
<body>
<h1>Welcome to My Simple Web Page</h1>
<p>This is a demonstration of how HTML, CSS, and JavaScript work together.</p>
<button onclick=”changeContent()”>Click Me</button>
<div id=”output”></div>
</body>
</html>
CSS (Style)
CSS enhances the visual appeal of your webpage. It helps style the text, layout, and button:
<style>
body {
font-family: Arial, sans-serif;
margin: 20px;
}
h1 {
color: #333;
}
button {
padding: 10px 20px;
background-color: #4CAF50;
color: white;
border: none;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
</style>
JavaScript (Interactivity)
JavaScript adds interactivity by dynamically changing content when the user clicks the button:
<script>
function changeContent() {
document.getElementById(‘output’).innerHTML = “You clicked the button!”;
}
</script>
SEO and Performance Benefits
- HTML plays a crucial role in SEO. Using semantic tags such as <header>, <article>, and <footer> helps search engines understand your content’s structure, improving ranking.
- CSS optimization for speed, such as minifying stylesheets and using efficient selectors, enhances page load time and mobile responsiveness.
- JavaScript should be lightweight and used sparingly to avoid impacting page performance. Deferring non-critical scripts can boost page speed, an important factor for SEO.
Interactive Example
Here’s an interactive coding example where you can modify CSS or JavaScript live and see how it changes the page in real-time:
<!DOCTYPE html>
<html lang=”en”>
<head>
<title>Interactive Example</title>
<style>
body {
font-family: Arial;
padding: 20px;
}
button {
padding: 10px 20px;
background-color: #007bff;
color: white;
border: none;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
.change {
color: red;
}
</style>
</head>
<body>
<h1 id=”heading”>Hello, World!</h1>
<button onclick=”document.getElementById(‘heading’).classList.toggle(‘change’)”>Change Style</button>
</body>
</html>
Learning Path
- Beginner: Start by mastering HTML tags, CSS properties (like colors, fonts, and layout), and basic JavaScript functions. Focus on the box model (margin, padding, border), basic styling, and simple DOM manipulation.
- Advanced: Dive into media queries for responsive design, Flexbox and CSS Grid for advanced layouts, and JavaScript’s ES6+ features like arrow functions, destructuring, and promises for async programming.
Real-World Application
Many popular websites like Airbnb and Amazon rely heavily on the combination of HTML, CSS, and JavaScript to create seamless, responsive user experiences. These platforms use advanced CSS techniques to ensure layouts work across different devices and JavaScript to handle everything from real-time data updates to user interactions.
Web Development Best Practices
Adhering to best practices in web development ensures that your web pages are accessible, maintainable, and performant. Here are some key practices to follow:
- Use Semantic HTML: Structure your web pages with meaningful HTML tags like <header>, <nav>, <article>, and <footer>. This not only improves accessibility but also helps search engines understand your content better.
- Organize Your CSS: Keep your CSS organized and modular by using techniques like CSS preprocessors (e.g., SASS, LESS) and CSS frameworks (e.g., Bootstrap). This makes your stylesheets easier to maintain and scale.
- JavaScript for Interactivity: Use JavaScript to add interactivity to your web pages, such as form validation, animations, and dynamic content updates. However, avoid using JavaScript for layout and styling, as this is best handled by CSS.
- Cross-Browser Compatibility: Test your web pages in multiple browsers and devices to ensure they work consistently. Tools like BrowserStack can help you automate this process.
- Version Control: Use version control systems like Git to manage your code and collaborate with other developers. This allows you to track changes, revert to previous versions, and work on different features simultaneously.
- Accessibility and Fallbacks: Follow web development best practices, such as using alt text for images and providing fallbacks for older browsers. This ensures that your web pages are accessible to all users, regardless of their device or browser.
- Continuous Learning: Stay up-to-date with the latest web development trends and technologies. Follow industry blogs, attend webinars, and participate in coding communities to continuously improve your skills.
SEO and Technical Benefits
Optimizing HTML for SEO
Using semantic HTML is essential for improving your website’s search engine rankings. Semantic HTML elements, such as <header>, <nav>, <article>, and <footer>, provide meaning to the content beyond mere structure. This helps search engines like Google understand the hierarchy and relevance of your page content, which in turn boosts your site’s SEO.
For example, using proper header tags (<h1>, <h2>, <h3>) for titles and subheadings creates a clear structure, making it easier for crawlers to index your page accurately. Adding structured data (e.g., schema.org) provides even more context, improving the likelihood of your site showing up in rich results like featured snippets.
SEO Impact of CSS
CSS contributes indirectly to SEO through page load speed and user experience, both of which are ranking factors. Lightweight CSS that’s minified and optimized for performance can significantly reduce page load time. This results in a better user experience and a higher ranking on search engines.
CSS can also be optimized by using external stylesheets and loading only the necessary CSS for each page. Techniques such as lazy loading and critical CSS (loading only the styles needed to render the first part of the page) ensure faster performance.
JavaScript and SEO
JavaScript adds interactivity and dynamic elements to a webpage, which can significantly improve user engagement. However, if not optimized correctly, JavaScript can hinder SEO efforts. Search engine crawlers might not fully execute JavaScript, leading to incomplete indexing of your pages.
To mitigate this, use techniques such as deferred loading or lazy loading of JavaScript. Ensure that critical content is accessible without requiring JavaScript, or use server-side rendering (SSR) to provide a fully rendered HTML page to crawlers. Additionally, consider using AJAX sparingly to load content dynamically, as improper use of AJAX can hide content from search engines.
Key Points for Optimizing JavaScript for SEO:
- Defer non-essential JavaScript to avoid blocking page rendering.
- Use progressive enhancement to ensure core content is available to crawlers without JavaScript.
- Implement SSR to provide a fully rendered version of JavaScript-heavy pages.
7. Advanced Techniques and Challenges
For advanced developers, optimizing for browser compatibility and performance is key to building scalable, efficient websites.
Key Challenges:
- Browser Compatibility: Different browsers render CSS and JavaScript differently, and ensuring consistent behavior across browsers can be challenging. Tools like Autoprefixer help automate cross-browser CSS compatibility.
- Performance Issues: As websites grow in complexity, loading times can suffer. Implementing code-splitting and lazy loading are effective ways to reduce JavaScript payloads and improve site speed.
- Best Practices for Scalable Code: Writing modular CSS and JavaScript, adhering to naming conventions (BEM), and using preprocessors (SASS/LESS) contribute to maintainable and scalable codebases.
8. Interactive Learning and Next Steps
To further develop your skills in HTML, CSS, and JavaScript, there are numerous platforms offering comprehensive learning paths for both beginners and advanced users.
Recommended Resources:
- FreeCodeCamp: Offers in-depth, hands-on coding challenges for all skill levels.
- Coursera’s Web Development Courses: Provides structured learning paths with a mix of theory and practical projects.
Explore interactive coding examples embedded in this guide to apply what you’ve learned. For advanced learners, try contributing to open-source projects or taking part in coding challenges on platforms like HackerRank or LeetCode.
Web Development Examples
Web development projects can range from simple web pages to complex web applications. Here are some examples to illustrate the versatility of web development:
- Simple Web Page: A basic web page built using HTML, CSS, and JavaScript might include a navigation menu, a hero image, and a call-to-action button. This type of project is ideal for beginners to practice the fundamentals of web development.
- Complex Web Application: A more advanced project might involve building a web application with a database, user authentication, and dynamic content generation. For example, an e-commerce site requires a robust backend to manage products, orders, and user accounts.
- Mobile and Progressive Web Apps: Web development also extends to building mobile apps and progressive web apps (PWAs). These applications offer a native app-like experience on the web, with features like offline access and push notifications.
- Personal Projects: Building a personal website, blog, or portfolio is a great way to showcase your skills and creativity. These projects allow you to experiment with different technologies and design patterns.
- Professional Projects: Working on larger projects, such as developing a web application for a company or organization, involves collaboration with other developers and stakeholders. These projects require a deep understanding of web development best practices and the ability to deliver high-quality, scalable solutions.
Finding Your Niche in Web Development
Mastering HTML, CSS, and JavaScript is essential for any web developer aiming to build high-performing, SEO-friendly websites. By learning how to optimize your code for both users and search engines, you’ll position yourself as a valuable developer in today’s digital landscape.
Take the next step by exploring advanced tutorials, joining web development communities, and contributing to open-source projects. Start building your own projects to hone your skills and make your mark in web development.
Conclusion
Web development is a complex and multifaceted field that requires a range of technical skills and knowledge. HTML, CSS, and JavaScript are the building blocks of the web, and mastering these technologies is essential for any web development project.
By following best practices and staying up-to-date with the latest trends and technologies, web developers can build fast, secure, and accessible web pages and applications. Web development is a creative and rewarding field that offers many opportunities for career advancement and personal growth.
Whether you’re just starting out or are an experienced web developer, there’s always more to learn and discover in the world of web development. Embrace the challenges, stay curious, and continue honing your skills to make your mark in this dynamic industry.